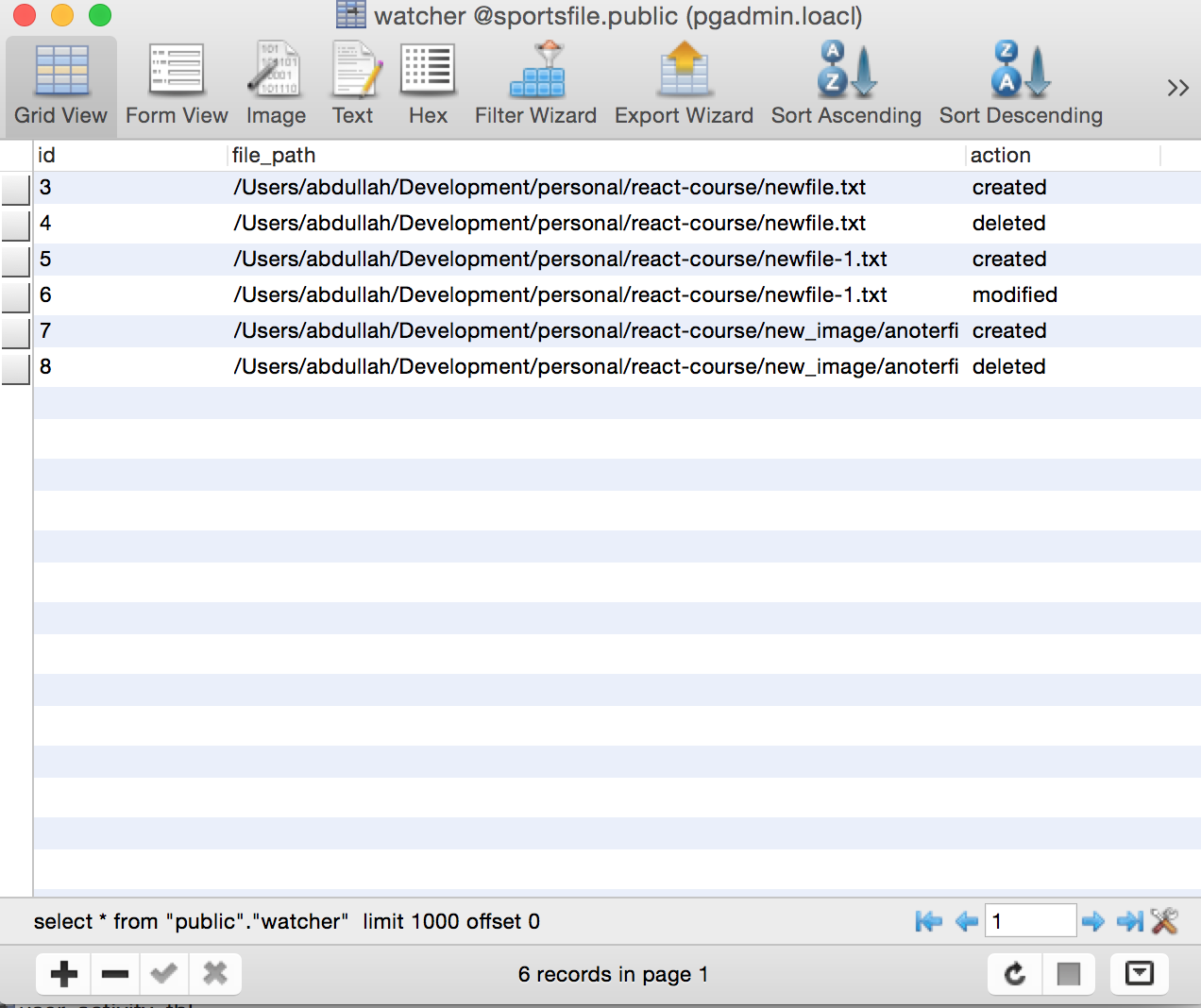
watchdog is a python package uses to notify linux kernel subsystem to watch for any changes to the filesystem. This is very handy package
Lets create a watcher using watchdog and save information to DB when any event occur.
1. Firstly I will write watcher class which look like
import time
import psycopg2
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
class Watcher:
DIRECTORY_TO_WATCH = "/Users/abdullah/Development/personal/watcher-folder"
def __init__(self):
self.observer = Observer()
def run(self):
event_handler = Processor() // This will handle all event from watchdog
self.observer.schedule(event_handler, self.DIRECTORY_TO_WATCH, recursive=True)
self.observer.start()
try:
while True:
time.sleep(5)
except:
self.observer.stop()
print "Error"
self.observer.join()
In this class Processor will be a new class which will process all event which is coming from watchdog observer
Let’s write Processor class
class Processor(FileSystemEventHandler):
@staticmethod
def on_any_event(event):
if event.is_directory:
return None
else:
# Save to DB.
Processor.save_to_db( event.src_path, event.event_type)
@classmethod
def save_to_db(cls, file_path, action):
try:
conn = psycopg2.connect(host="localhost",database="watcher_db", user="admin", password="123456")
sql = """INSERT INTO watcher(file_path, action)
VALUES(%s, %s);"""
cur = conn.cursor()
# execute the INSERT statement
cur.execute(sql, (file_path,action))
conn.commit()
# close communication with the database
cur.close()
except (Exception, psycopg2.DatabaseError) as error:
print(error)
finally:
if conn is not None:
conn.close()
In the Processor class method on_any_event received event from observer when any event occurs on watching directory. I have skipped event processing when any directory changes. event will be processed or saved to DB only file event occurs like ‘created’, ‘modified’, ‘deleted’
So, let’s add final block to run this script
if __name__ == '__main__':
w = Watcher()
w.run()
** Before run this script you need to add table ‘watcher’ with column (id, file_path, action)
now let’s run script using
python watcher.py
if you create any files on watching directory you will get that information to DB
To actually make this useful, you should wrap this up as a daemon or upstart script which can be run indefinitely.